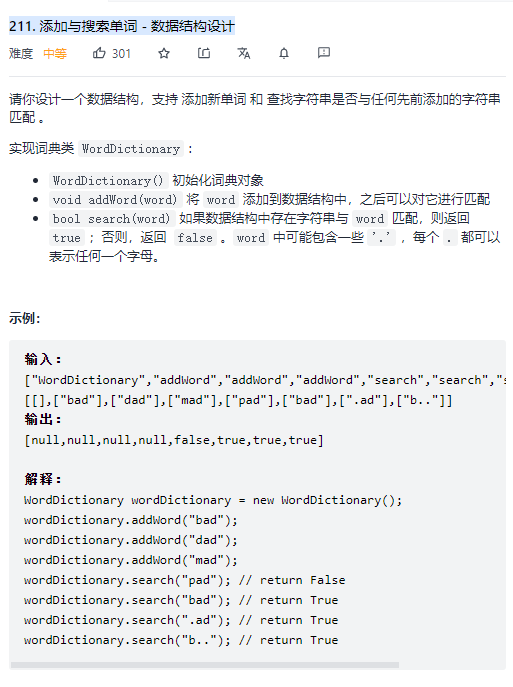
解题思路:
比较典型的一道前缀树的题目,注意在构建前缀树的过程中不要粗心大意即可,前缀树的定义可以参考208. 实现 Trie (前缀树) 的官方题解。
1.用一个is_end来记录是否到达结尾,2.结点向下的多叉树子结点用vector<TrieNode *>来记录
以下是C++代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65
| class TrieNode{ public: vector<TrieNode *> childs; bool is_end;
TrieNode(bool _is_end=false){ childs=vector<TrieNode *>(26,nullptr); is_end=_is_end; }
};
class WordDictionary { public: WordDictionary() { head_node=new TrieNode(false); } void addWord(string word) { int length=word.length(); TrieNode *temp=head_node; for(int i=0;i<length;i++){ char ch=word[i]; int index=ch-'a'; if (temp->childs[index]==nullptr){ temp->childs[index]=new TrieNode(); } temp=temp->childs[index]; } temp->is_end=true;
} bool search(string word) { return search(word,0,head_node); }
bool search(string word,int index,TrieNode* node){ if (index==word.length()){ if (node->is_end) return true; else return false; } char ch=word[index]; if (ch!='.'){ int index_=ch-'a'; TrieNode* temp=node->childs[index_]; if(temp!=nullptr&&search(word,index+1,temp)){ return true; } }else{ for(int i=0;i<26;i++){ TrieNode* temp=node->childs[i]; if(temp!=nullptr&&search(word,index+1,temp)){ return true; } } } return false; } private: TrieNode *head_node; };
|